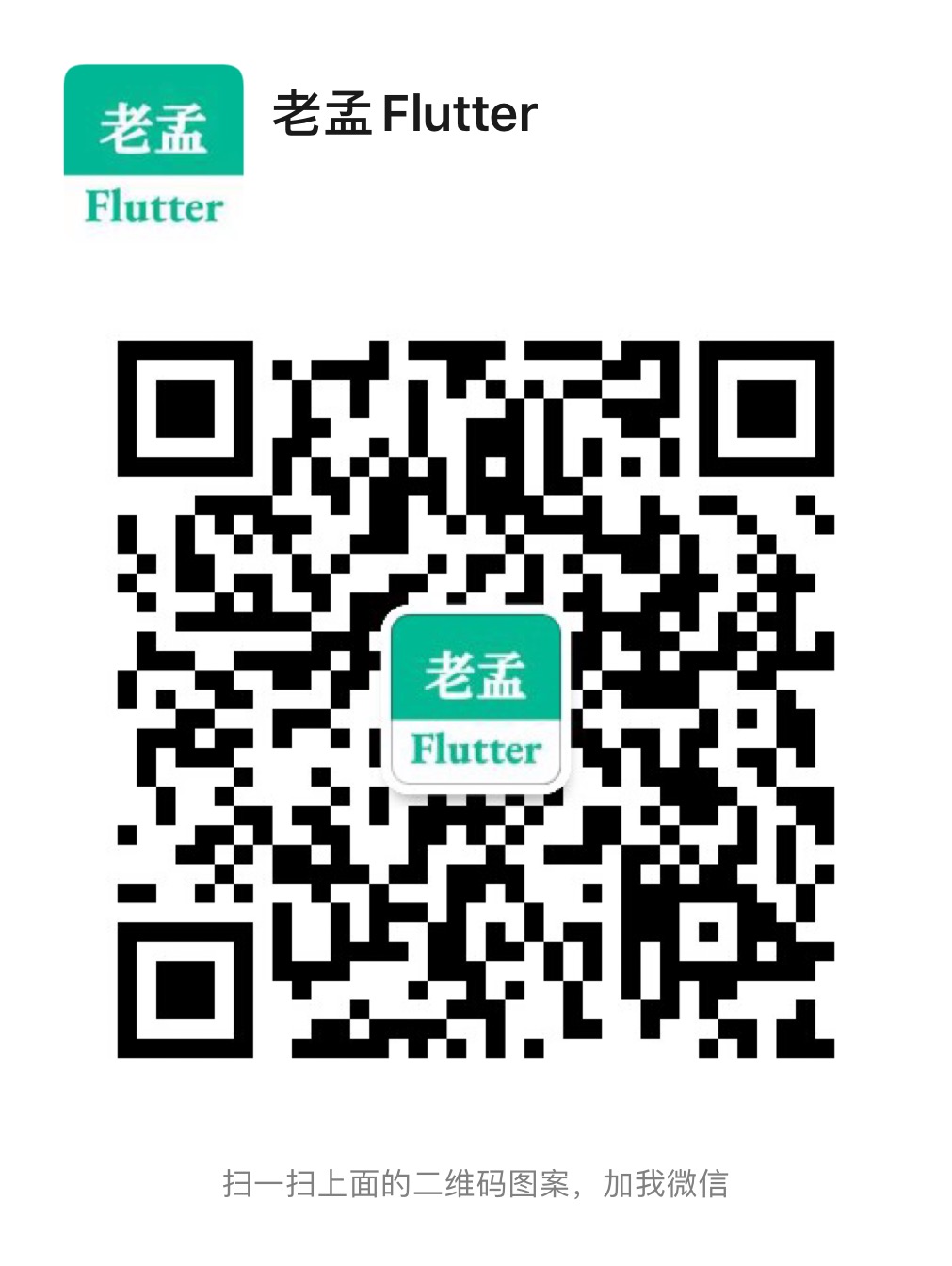
微信交流群
半圆菜单展开/收起代码如下:
import 'dart:math';
import 'package:flutter/material.dart';
class DemoFlowMenu extends StatefulWidget {
_DemoFlowMenuState createState() => _DemoFlowMenuState();
}
class _DemoFlowMenuState extends State<DemoFlowMenu>
with TickerProviderStateMixin {
//动画需要这个类来混合
//动画变量,以及初始化和销毁
AnimationController _ctrlAnimationCircle;
void initState() {
super.initState();
_ctrlAnimationCircle = AnimationController(
//初始化动画变量
lowerBound: 0,
upperBound: 80,
duration: Duration(milliseconds: 300),
vsync: this);
_ctrlAnimationCircle.addListener(() => setState(() {}));
}
void dispose() {
_ctrlAnimationCircle.dispose(); //销毁变量,释放资源
super.dispose();
}
//生成Flow的数据
List<Widget> _buildFlowChildren() {
return List.generate(
5,
(index) => Container(
child: Icon(
index.isEven ? Icons.timer : Icons.ac_unit,
color: Colors.primaries[index % Colors.primaries.length],
),
));
}
Widget build(BuildContext context) {
return Stack(
children: <Widget>[
Positioned.fill(
child: Flow(
delegate: FlowAnimatedCircle(_ctrlAnimationCircle.value),
children: _buildFlowChildren(),
),
),
Positioned.fill(
child: IconButton(
icon: Icon(Icons.menu),
onPressed: () {
setState(() {
//点击后让动画可前行或回退
_ctrlAnimationCircle.status == AnimationStatus.completed
? _ctrlAnimationCircle.reverse()
: _ctrlAnimationCircle.forward();
});
},
),
),
],
);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
FlowAnimatedCircle 代码如下:
class FlowAnimatedCircle extends FlowDelegate {
final double radius; //绑定半径,让圆动起来
FlowAnimatedCircle(this.radius);
void paintChildren(FlowPaintingContext context) {
if (radius == 0) {
return;
}
double x = 0; //开始(0,0)在父组件的中心
double y = 0;
for (int i = 0; i < context.childCount; i++) {
x = radius * cos(i * pi / (context.childCount - 1)); //根据数学得出坐标
y = radius * sin(i * pi / (context.childCount - 1)); //根据数学得出坐标
context.paintChild(i, transform: Matrix4.translationValues(x, -y, 0));
} //使用Matrix定位每个子组件
}
bool shouldRepaint(FlowDelegate oldDelegate) => true;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
版权所有,禁止私自转发、克隆网站。