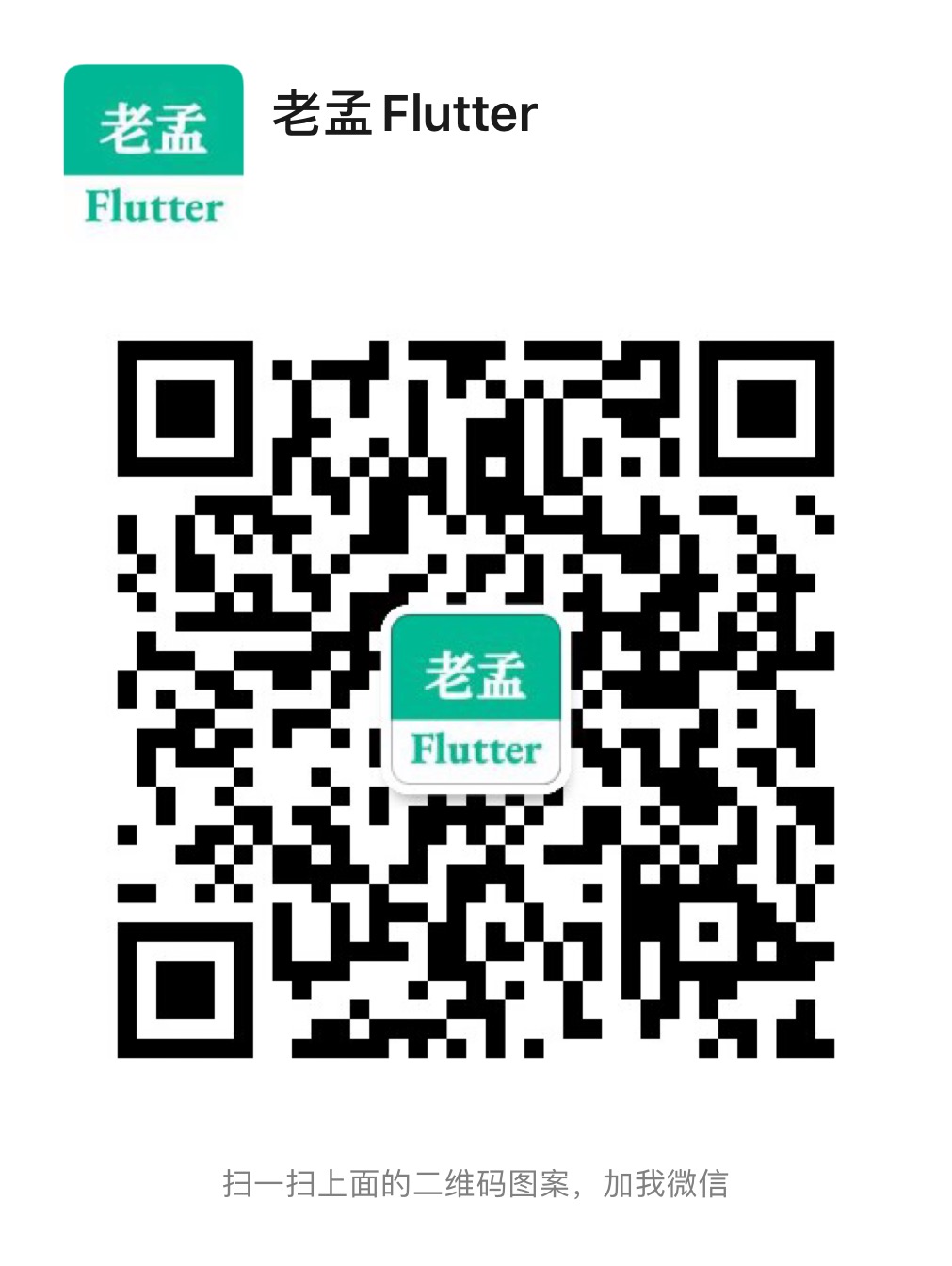
微信交流群
# 仿 掘金-我 效果
先看下效果:
拿到效果图先不要慌 (取出手机拍照发个朋友圈😊),整个列表每一行的布局基本一样,所以先写出一行的效果:
class _SettingItem extends StatelessWidget {
const _SettingItem(
{Key key, this.iconData, this.iconColor, this.title, this.suffix})
: super(key: key);
final IconData iconData;
final Color iconColor;
final String title;
final Widget suffix;
Widget build(BuildContext context) {
return Container(
height: 45,
child: Row(
children: <Widget>[
SizedBox(
width: 30,
),
Icon(iconData,color: iconColor,),
SizedBox(
width: 30,
),
Expanded(
child: Text('$title'),
),
suffix,
SizedBox(
width: 15,
),
],
),
);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
消息中心和其他行最后的样式不一样,单独封装,带红色背景的组件:
class _NotificationsText extends StatelessWidget {
final String text;
const _NotificationsText({Key key, this.text}) : super(key: key);
Widget build(BuildContext context) {
return Container(
padding: EdgeInsets.symmetric(horizontal: 10),
decoration: BoxDecoration(
shape: BoxShape.rectangle,
borderRadius: BorderRadius.all(Radius.circular(50)),
color: Colors.red),
child: Text(
'$text',
style: TextStyle(color: Colors.white),
),
);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
灰色后缀组件:
class _Suffix extends StatelessWidget {
final String text;
const _Suffix({Key key, this.text}) : super(key: key);
Widget build(BuildContext context) {
return Text(
'$text',
style: TextStyle(color: Colors.grey.withOpacity(.5)),
);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
将这些封装好的组件组合起来:
class SettingDemo extends StatelessWidget {
Widget build(BuildContext context) {
return Column(
children: <Widget>[
_SettingItem(
iconData: Icons.notifications,
iconColor: Colors.blue,
title: '消息中心',
suffix: _NotificationsText(
text: '2',
),
),
Divider(),
_SettingItem(
iconData: Icons.thumb_up,
iconColor: Colors.green,
title: '我赞过的',
suffix: _Suffix(
text: '121篇',
),
),
Divider(),
_SettingItem(
iconData: Icons.grade,
iconColor: Colors.yellow,
title: '收藏集',
suffix: _Suffix(
text: '2个',
),
),
Divider(),
_SettingItem(
iconData: Icons.shopping_basket,
iconColor: Colors.yellow,
title: '已购小册',
suffix: _Suffix(
text: '100个',
),
),
Divider(),
_SettingItem(
iconData: Icons.account_balance_wallet,
iconColor: Colors.blue,
title: '我的钱包',
suffix: _Suffix(
text: '10万',
),
),
Divider(),
_SettingItem(
iconData: Icons.location_on,
iconColor: Colors.grey,
title: '阅读过的文章',
suffix: _Suffix(
text: '1034篇',
),
),
Divider(),
_SettingItem(
iconData: Icons.local_offer,
iconColor: Colors.grey,
title: '标签管理',
suffix: _Suffix(
text: '27个',
),
),
],
);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
版权所有,禁止私自转发、克隆网站。